Table of Contents
Before we begin, I must talk about what I mean by editing, and what I intend to cover. I believe that the most important thing about editing is code completion. In this section I pay special attention to python although PyCharm offers superb code completion for other languages like SQL and JavaScript.[1]
I start off with code completion, because if you're like me, you have little time and want to dive into the thick of things and code completion is what we want most out of an IDE.
Then, I talk about a couple of editor settings that help keep me sane.
Code Completion
PyCharm's editor is very powerful and extensible. In this section we will go over some useful tools that I use to write less error prone code faster.
Smart Completion
You get suggestions as you type. You can further enhance this by the following:
- Collecting runtime information
- Adding docstrings to functions and methods
- Invoking
assert
statements
Runtime Information
Lets start with the easiest enhancement. All we need to do is check this box, and PyCharm will give you better code completion as you debug your program more:
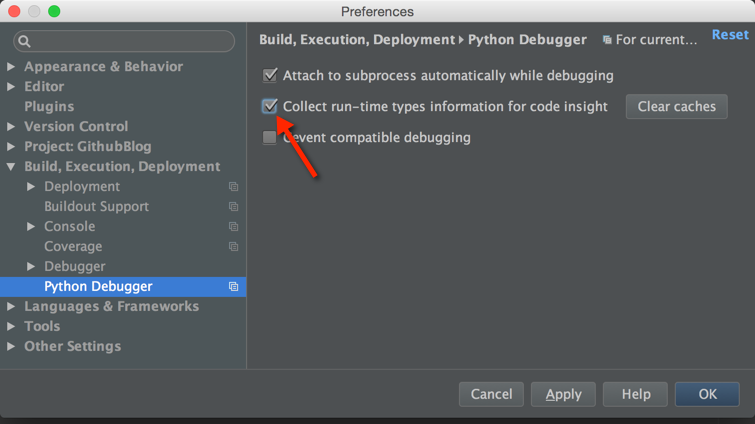
If you ever get wrong suggestions for completion clear the caches. Running your tests in debug mode will also give PyCharm a better chance to get an understanding of the types that you're using.
Docstrings
Writing docstrings helps PyCharm, but also helps developers gain a better understanding of the code that you have written. PyCharm understands docstrings and uses the information in them to give you code completion and give you warning signals if it thinks you're being inconsistent:
def foo(a, b, c):
"""
:param a: A for apple
:type a: str
:param b: B for ball
:type b: str
:param c: C for cat
:type c: str
:return: The answer to everything is 42. Remember ye well!
:rtype: int
"""
return 42
if __name__ == '__main__':
foo(1, 2, 3)
In the example above, I've used PyCharm tells me that I'm being inconsistent:
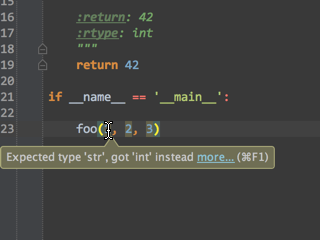
But there's more! If you wrote docstrings, then PyCharm will give you a beautiful documentation popup about your variables (by using quick documentation):[2]
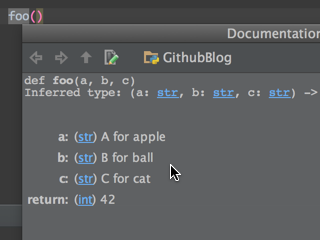
Assert Statements
assert
statements can help tell PyCharm what types you should expect. Please note that, assert
statements are not overhead free, unlike docstrings. I would advise using them in tests, so that the debugger can collect type information about your parameters. For example, the following will give code completions for a
but not for b
:
def foo(a, b, c):
assert isinstance(a, str)
return 42
This is because PyCharm knows about a
, and that its a string, but it doesn't know about b
:
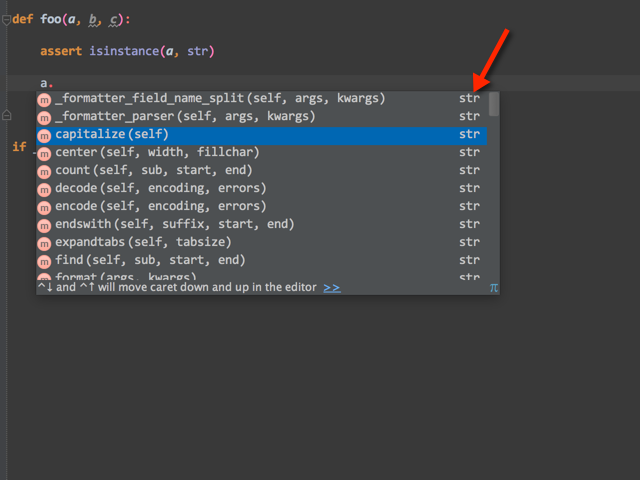
Hippie Complete
Hippie Complete or Cyclic completion is best explained with a video:
Basically, it completes the word based on previous words you've typed in the same document.
Spelling Correction
PyCharm also offers spelling correction as a quick fix. If you misspell "massachussets" like so:
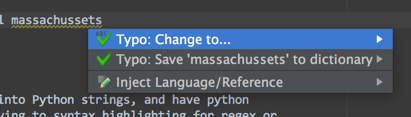
Then you can try quick fixing it with ⌥ + return. After you hit return on the first option above, you should see correction options:
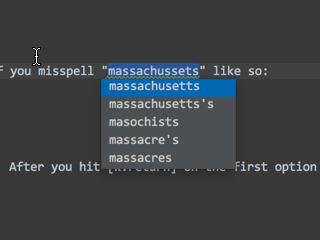
This was a request on reddit
Language Injection
Language Inject basically means that you can inject other languages into Python strings, and have python recognize those languages. This comes in really handy when you're trying to syntax highlighting for regex or html, but it goes further than that often providing code completion. In order to get language injection, one must invoke the quick fix command inside string delimiters (''
).[2]
Here is a video to demonstrate how language injection works:[3]
Editor
This part covers the settings regarding the editor for better workflow. The options I set are based on my own preferences and hence not scientifically tested but they do make my editing a lot faster. Take the following advice with a healthy dose of skepticism since what might work for me, might not work for you.
Font
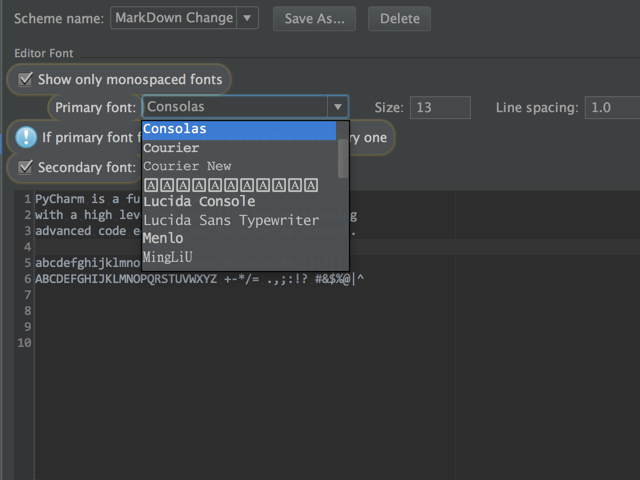
Consolas is the only font that looks good on Windows, Mac and Linux. Consolas remains monospace regardless of whether it is bold or italic, and the only font that looks consistently good in PyCharm. I have tested this on Mac OSX Yosemite, Windows 8.1 and Ubuntu (14.04).[4]
If you've had success with other fonts (especially in Linux), please do mention them in the comments.
Braces and Quotes
A lot of the time, I forget to put parentheses around things or quotes around strings. By enabling the following option, you can select text, and when you press " or ' or ( or ), instead of replacing the text with the character entered, it will surround the selection with quotes or parentheses:
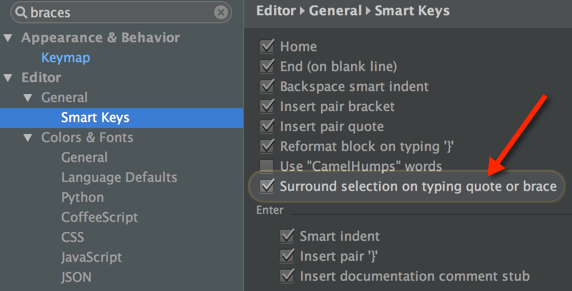
Case Sensitive Completion
By default, PyCharm comes with first letter case sensitivity, meaning that if you were to type e
, Exception
would not be offered as a viable completion. This is why I disable case sensitivity:
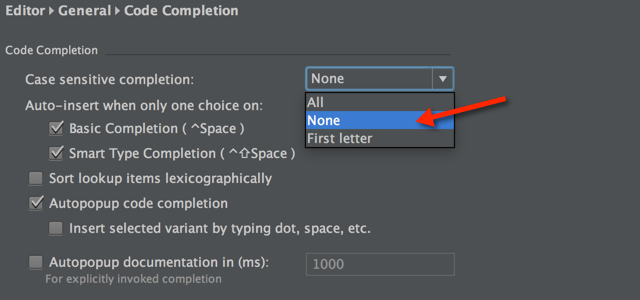
Please note however, that on slower machines this does make code completion slower.
Up Next
I will likely be covering how to deal with interpreters.
Previously
I talked about a couple of things in part one.
Update
2015-01-19 11:41 : Added a section on Spelling Correction
[1] | I will cover javascript, html and other stuff in another section that deals with web tools. Don't worry I know that they are indispensable tools, and will make sure to give them the time they deserve. |
[2] | (1, 2) Use the keymap to find your corresponding keyboard shortcut discussed in the previous part. |
[3] | Don't worry if you don't know what emmet does, I'll cover that when I cover web tools. |
[4] | Take a look at this answer if you want to use consolas on Ubuntu: |